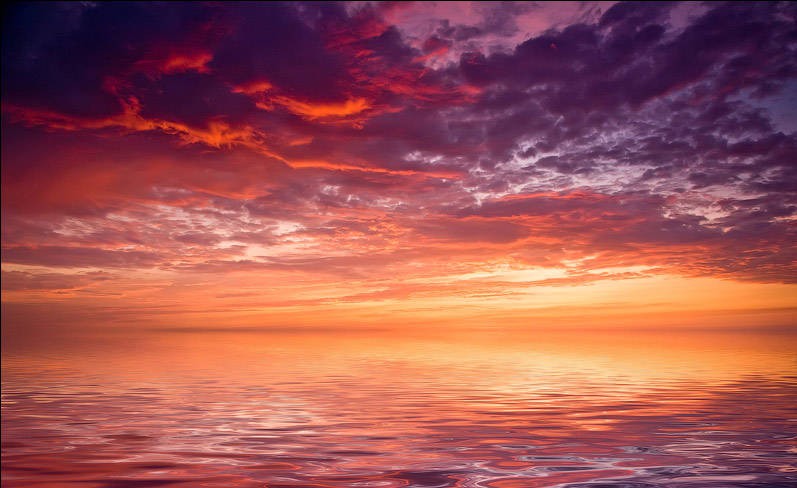
First go
I have decided to have a serious go at learning Go. I’ve looked at the language for a while and read a couple of books about it, but now the time has come to get stuck in and code a real live project for myself. I want the project to be a time and task management tool that helps me (and me team) to collaborate on a common task list. It will have a JavaScript & HTML client program along with a series of server-side REST APIs that control access to a PostgresSQL database back-end.
First things first I need to decide if I want to code in my text editor sublime or if I would prefer to code in an IDE of some sort. I looked into the ‘gosublime’ plugin for my text editor and it no longer seems to be available in the package control menu. I see a bunch of other plug-ins for golang and various Go tools, but given the lack of direction and documentation from Sublime, I decided that perhaps a purpose built Go IDE would be a better choice.
I reviewed offerings from Eclipse (goclipse), and Intellij’s GoLand, but they both suffered from the same bloat they inherited from their parent products. I finally chanced upon an IDE called LiteIDE that seems to have everything I’m looking for, insofar as it is lightwieght, not encumbered by bloat due to being overly generic, it’s task-specific and focused on Go.
Installing LiteIDE 37.1
I downloaded and installed LiteIDE from the 37.1 LiteIDE release folder on git hub. I went for the liteidex37.1.linux64-qt5.5.1-system.tar.gz as I am installing on Ubuntu 20.04. I figured out which version to go for by executing ‘qtchooser -l’ on the console to see which versions of Qt come pre-installed on this version of Ubuntu.
richard@Valhalla:~$ qtchooser -l
4
5
default
qt4-x86_64-linux-gnu
qt4
qt5-x86_64-linux-gnu
qt5
Given that I have both Qt 4 and Qt 5 available, I opted for the later of the two.
As for the install, I simply unpacked the tar.gz file and placed it’s contents in a convenient location for me. Then I symlinked the liteide binary from the LiteIDE bin folder into my ~/bin directory so I can launch liteide from the console.
First program
Once I had LiteIDE up and running, I made a new ‘command’ project and put the following code into it:
package main
import (
"log"
"net/http"
)
type server struct{}
func (s *server) ServeHTTP(w http.ResponseWriter, r *http.Request) {
w.Header().Set("Content-Type", "application/json")
w.WriteHeader(http.StatusOK)
w.Write([]byte(`{"message": "wooga"}`))
}
func main() {
s := &server{}
http.Handle("/", s)
log.Fatal(http.ListenAndServe(":8080", nil))
}
I’m now able to browse to http://localhost:8080 and see my crusty JSON document! So far so good… now I need to find out the best practices for developing REST APIs with Go.