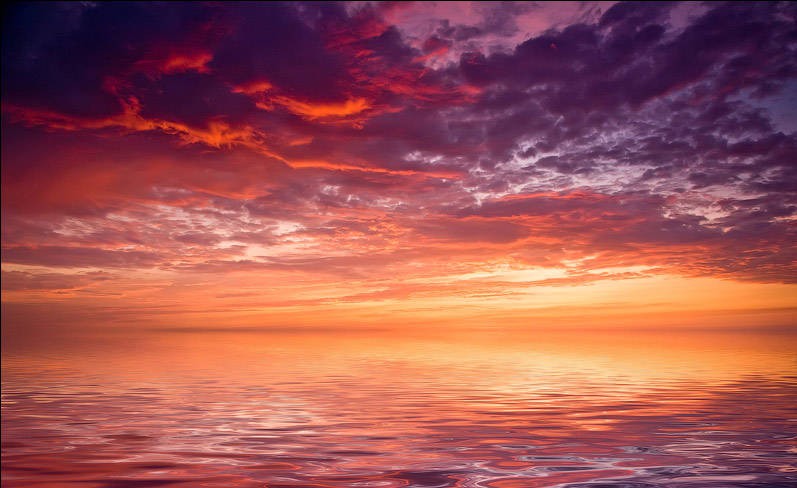
Debounce
In electronics when you close a switch or connect a pair of wires together, you get a ripple of current that forms as the connection is coming together known as Contact Bounce this bounce or chatter can manifest itself as a number of closely spaced interrupts that can ruin your day as a programmer if they’re not supressed.
Likewise, in any system that generates a bunch of events your code needs to respond to as a group, you need a debounce function. Here is an example written in JavaScript.
function debounce(delay, callback) {
let timeout;
return function() {
clearTimeout(timeout);
timeout = setTimeout(callback, delay);
}
}
A classic implementation example of debounce() is a type-ahead search box where you want the user to enter some text and then use the text they entered to conduct a search on a back-end database. Sending a search query to the back-end with each keystroke the user makes, puts undue (and needless) pressure on the back-end database. So we use a debounce to only send the search to the back-end once the user stops typing for a moment. e.g.
var searchBox = document.getElementById("searchBox");
searchBox.addEventListener("keyup", debounce(250, function(){
// fetch some stuff from the database here
}));
So we can see in the above example, if the user waits for 250ms or more, then the code will send the request to the back-end. However, if the user keeps typing then the debounce function will keep resetting it’s timeout until the user pauses input for at least 250ms.