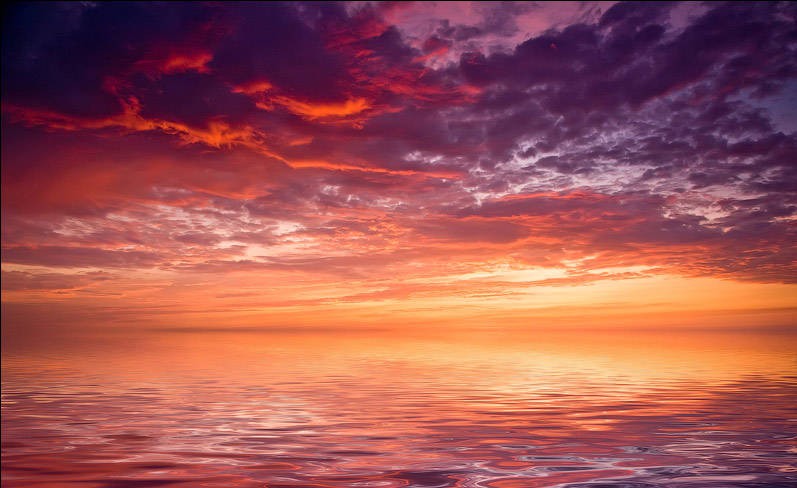
Coding cruddy...
A couple of years ago I wrote an open source library called cruddy that I used to provide a simple data access layer. It's mission was to painlessly to convert objects into database records and back.
You use it like this...
public int update(SomeObject obj) throws SQLException {
try (Database db = Database.connect(connection)) {
// Save some object (called obj) to the database
int rows = db.update(obj);
return rows;
} catch (SQLException e) {
// handle errors here as per usual
}
}
Here's is an example of an object that can be saved to a database...
@Table(name = "organization")
public class Organization {
@PrimaryKey(isSerial = false)
private UUID id = UUID.randomUUID();
@Unique
@NotNull
private String name;
@Ignore
private List<OrganizationType> types = new ArrayList<>();
@JsonField
private Map<String, String> profile = new HashMap<>();
public Organization() {
}
public UUID getId() {
return id;
}
public void setId(UUID id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public List<OrganizationType> getTypes() {
return types;
}
public void setTypes(List<OrganizationType> types) {
this.types = types;
}
public Map<String, String> getProfile() {
return profile;
}
public void setProfile(Map<String, String> profile) {
this.profile = profile;
}
}
A series of annotations allow the coder to mark up a class so that it can be easily persisted to the database. Notable are the @Table, and @PrimaryKey annotations as these are mandatory. There are also annotations to support field name mapping, database constraints, foreign keys etc.
I wrote this handy little library because I was saddened by the complexity, brittleness and performance of tools like Hibernate. It's served me well as the heart of a number of commercial projects I've used it on.